API Methods
To create a payment you will use the callService method. It follows the flow pattern below. You start by making the request, then you will receive an acknowledgement, and later a callback with the updated status of your transaction.
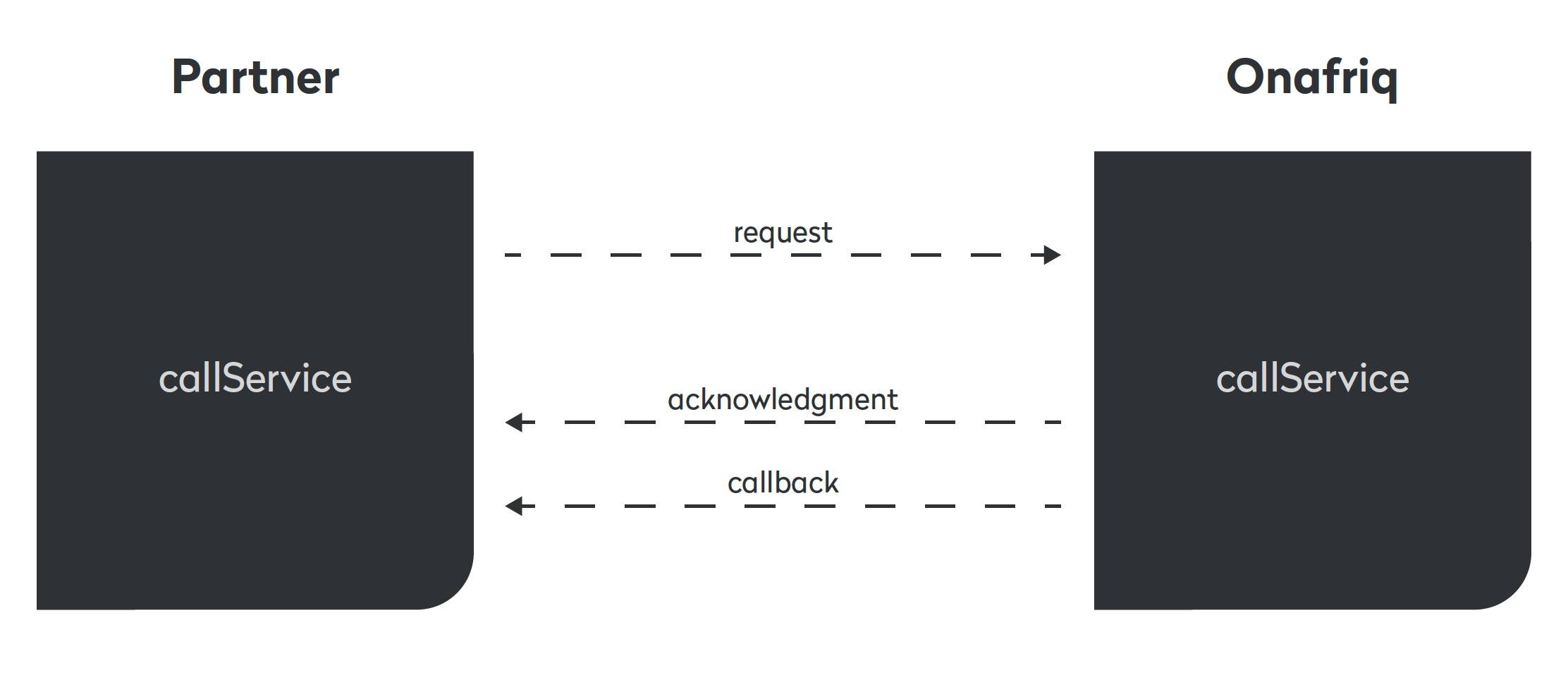
callService flow
Available Methods
Method | Description | Return value |
---|---|---|
callService | Create a transaction | ACK, and Webhook |
transactionStatus | Method sent from Onafriq to the Partner via the webhook to return the final status of the transaction created by callService | Status of transactions created by callService. See full list of status codes here. |
See below for method details
callService Request
Use this method to create a transaction.
{
"corporateCode": "",
"password": "",
"mfsSign": "",
"batchId": "Wayodi7",
"requestBody": [
{
"instructionType": {
"destAcctType": 1,
"amountType": 1
},
"amount": {
"amount": 2,
"currencyCode": "UGX"
},
"sendFee": {
"amount": 1,
"currencyCode": "UGX"
},
"sender": {
"msisdn": "",
"fromCountry": "GB",
"name": "Wys",
"surname": "Katlego",
"address": null,
"city": null,
"state": null,
"postalCode": null,
"email": null,
"dateOfBirth": null,
"document": null
},
"recipient": {
"msisdn": "",
"toCountry": "UG",
"name": "Gg",
"surname": "Lebese",
"address": null,
"city": null,
"state": null,
"postalCode": null,
"email": null,
"dateOfBirth": null,
"document": null,
"destinationAccount": null
},
"thirdPartyTransId": "MFS11",
"reference": null
}
]
}
Parameters
Field | Type | Mandatory | Description | Comment |
---|---|---|---|---|
corporateCode | String | Yes | Unique corporate code | Will be provided by Onafriq. |
password | String | Yes | Secure password | Will be provided by Onafriq. |
mfsSign | String | Yes | MFS signature | Message Level Security |
batchId | String | Yes | Sender batch ID. | |
instructionType: | ||||
destAcctType | Integer | Yes | Defines remittance destination as either to Mobile Wallet or Bank account | Valid values: 1 = Mobile Wallet 2 = Bank |
amountType | Integer | Yes | Defines amount as either Send amount in send currency or Receive amount in receive currency. | Valid values: 1 = Send amount 2 = Receive (payout) amount |
amount: | ||||
amount | Numeric | Yes | Instruction amount | |
currencyCode | String | Yes | Instruction currency. Currency code (ISO 4217) | |
sendFee: | ||||
amount | Numeric | Optional | Sending fee amount | Required when passing instruction with amount type ‘’Send’’. |
currencyCode | String | Optional | Fee currency code (ISO 4217) | Required when passing instruction with amount type ‘’Send’’. |
sender: | ||||
msisdn | String | No | Sender mobile number passed in ITU-T E.164 GSM DCS 1800 format, e.g. 250700800900. | May be passed as empty if sender has no mobile phone. |
fromCountry | String | Yes | Send country (ISO 3166-2) | |
name | String | Yes | Sender first name | |
surname | String | Yes | Sender surname | |
address | String | No | Sender address | |
city | String | No | City | |
state | String | No | State | |
postalCode | String | No | Postal code | |
String | No | Sender email | ||
dateOfBirth | date | No | Sender date of birth; YYYY-MM-DD | |
document: | ||||
idNumber | String | No | Sender ID number | |
idType | String | No | Identification Type | Possible values: PP = Passport ID = National ID card) DL = Driver’s License |
idCountry | String | No | Sender ID country/nationality | |
idExpiry | date | No | Expiry date of sender ID; YYYY-MM-DD | |
recipient: | ||||
msisdn | String | Conditional | Recipient mobile number passed in ITU-T E.164 GSM DCS 1800 format, e.g. 250700800900 | Required where dest_account_type is mobile wallet. |
toCountry | String | Yes | Destination country (ISO 3166-2) | |
name | String | Yes | Recipient first name | |
surname | String | Yes | Recipient surname | |
address | String | No | Recipient address | |
city | String | No | City | |
state | String | No | State | |
postalCode | String | No | Postal code | |
String | No | Recipient email | ||
dateOfBirth | date | No | Recipient date of birth; YYYY-MM-DD | |
document: | ||||
idNumber | String | No | Recipient ID number | |
idType | String | No | Identification type | Possible values: PP = Passport ID = National ID card) DL = Driver’s License |
idCountry | String | No | Recipient ID country/nationality | |
idExpiry | date | No | Expiry date of Recipient ID; YYYY-MM-DD | |
destinationAccount: | ||||
accountNumber | String | Conditional | Recipient account number. May be mobile wallet account or bank account | |
mfsBankCode | String | Conditional | Unique reference for the bank on the Onafriq system | Required where the destination account type is a bank account |
thirdPartyTransId | String | Yes | Transaction ID of the sending 3P | |
reference | 1000 chars | No | A pass-through field that can be used to pass any other relevant detail. | Supports comma-separated values, if multiple details are being passed. |
Cash pickup reference values
If doing a cash pick-up transaction, see Cash Pickup References for the reference values to use.
callService Response
This is the response returned by the callService method
{
"totalTxSent": 4,
"noTxAccepted": 4,
"noTxRejected": 0,
"details": {
"transResponse": [
{
"thirdPartyId": "MFS11",
"status": {
"code": "100",
"message": "Accepted"
}
},
{
"thirdPartyId": "MFS12",
"status": {
"code": "100",
"message": "Accepted"
}
},
{
"thirdPartyId": "MFS13",
"status": {
"code": "100",
"message": "Accepted"
}
},
{
"thirdPartyId": "MFSBank12",
"status": {
"code": "100",
"message": "Accepted"
}
}
]
},
"timestamp": "2019-12-18 07:50:26.771"
}
Parameters
Field | Type | Description |
---|---|---|
totalTxSent | Numeric | Total number of transaction submitted. |
noTxAccepted | Numeric | Total number of transaction that passed validation. |
noTxRejected | Numeric | Total number of transaction that failed validation. |
details: | Object | Object containing a list of transResponses |
transResponse | List | Details of transactions that passed or failed validation stage |
thirdPartyId | String | Transaction ID of the sending 3P |
status: | Object | Status Object containing status code, message and/or messageDetail |
code | String | Response status code. See list of codes here |
message | String | Response status message |
messageDetail | String | Detailed message when transaction fails |
timestamp | DateTime | Current TimeStamp for the list/batch sent |
callService Callback
Below is the format of the callback notification.
{
"thirdPartyTransId": "Test11",
"mfsTransId": "1126231250437",
"e_trans_id": "11524180437",
"fxRate": 3720.765,
"status": {
"code": "MR101",
"message": "success"
},
"sendAmount": {
"amount": 0,
"currencyCode": "UGX"
},
"receiveAmount": {
"amount": 2000,
"currencyCode": "UGX"
}
}
Parameters
Field | Type | Description |
---|---|---|
thirdPartyTransId | String | Transaction ID of the sending partner |
mfsTransId | String | MFS transaction Id |
e_trans_id | String | Transaction ID of the receiving platform. In the case of cash pick-up remittances, this value will be the voucher number. |
fxRate | Numeric | Value of the exchange rate |
status | Object | Status Object containing status code and message |
code | String | Response status code of transaction. See list of codes here |
message | String | Response status message of transaction |
sendAmount | Object | Object containing send amount and send currency |
amount | Numeric | Send amount |
currencyCode | String | Send currency code(ISO 4217) |
receiveAmount | Object | Object containing receive amount and receive currency |
amount | Numeric | receive amount |
currencyCode | String | receive currency code (ISO 4217) |
Updated 6 months ago